mirror of
https://github.com/meshtastic/firmware.git
synced 2025-05-01 11:42:45 +00:00
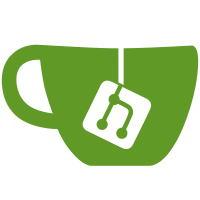
using @girtsf clang-format prefs settings. This should allow us to turn on auto format in our editors without causing spurious file changes.
47 lines
755 B
C++
47 lines
755 B
C++
#pragma once
|
|
|
|
#include <freertos/FreeRTOS.h>
|
|
#include <freertos/semphr.h>
|
|
|
|
namespace meshtastic
|
|
{
|
|
|
|
// Simple wrapper around FreeRTOS API for implementing a mutex lock.
|
|
class Lock
|
|
{
|
|
public:
|
|
Lock();
|
|
|
|
Lock(const Lock &) = delete;
|
|
Lock &operator=(const Lock &) = delete;
|
|
|
|
/// Locks the lock.
|
|
//
|
|
// Must not be called from an ISR.
|
|
void lock();
|
|
|
|
// Unlocks the lock.
|
|
//
|
|
// Must not be called from an ISR.
|
|
void unlock();
|
|
|
|
private:
|
|
SemaphoreHandle_t handle;
|
|
};
|
|
|
|
// RAII lock guard.
|
|
class LockGuard
|
|
{
|
|
public:
|
|
LockGuard(Lock *lock);
|
|
~LockGuard();
|
|
|
|
LockGuard(const LockGuard &) = delete;
|
|
LockGuard &operator=(const LockGuard &) = delete;
|
|
|
|
private:
|
|
Lock *lock;
|
|
};
|
|
|
|
} // namespace meshtastic
|