mirror of
https://github.com/meshtastic/firmware.git
synced 2025-04-23 17:13:38 +00:00
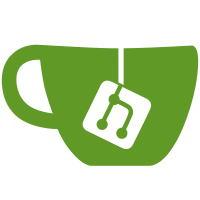
* Make STM compile again and update toolchain. The binary is too big for the flash. WIP * Making progress with OSFS, still WIP * more progress, still too big. Adding RAK3172 to the equasion * Make STM compile again and update toolchain. The binary is too big for the flash. WIP * Making progress with OSFS, still WIP * more progress, still too big. Adding RAK3172 to the equasion * still too big * minimize build * trunk fmt * fix a couple of symbol clashes * trunk fmt * down to 101% with a release vs. debug build and omitting the flash strings * fix compilation * fix compilation once more * update protobufs linkage * - Toolchain updated - Fixed macro error * silence compiler warning note: do something about this assert... * new toolkit and fix Power.cpp * STM32WL make it fit (#4330) * Add option to exclude I2C parts The I2C hals and related code uses a significant amount of flash space and aren't required for a basic node. * Add option to disable Admin and NodeInfo modules Disabled by default in minimal build. This saves a significant amount of flash * Disable unused hals These use up significant flash * Add float support for printf for debugging Makes serial look nice for debugging * This breaks my build for some reason * These build flags can save a bit of flash * Don't disable NodeInfo and Admin modules in minimal build They fit in flash * Don't include printf float support by default Only useful for debugging --------- Co-authored-by: Adam Lawson <dev@goshawk22.uk> --------- Co-authored-by: Ben Meadors <benmmeadors@gmail.com> Co-authored-by: Adam Lawson <dev@goshawk22.uk>
68 lines
1.8 KiB
C++
68 lines
1.8 KiB
C++
#undef RNG
|
|
#include "AES.h"
|
|
#include "CTR.h"
|
|
#include "CryptoEngine.h"
|
|
#include "configuration.h"
|
|
|
|
class STM32WLCryptoEngine : public CryptoEngine
|
|
{
|
|
|
|
CTRCommon *ctr = NULL;
|
|
|
|
public:
|
|
STM32WLCryptoEngine() {}
|
|
|
|
~STM32WLCryptoEngine() {}
|
|
|
|
virtual void setKey(const CryptoKey &k) override
|
|
{
|
|
CryptoEngine::setKey(k);
|
|
LOG_DEBUG("Installing AES%d key!\n", key.length * 8);
|
|
if (ctr) {
|
|
delete ctr;
|
|
ctr = NULL;
|
|
}
|
|
if (key.length != 0) {
|
|
if (key.length == 16)
|
|
ctr = new CTR<AES128>();
|
|
else
|
|
ctr = new CTR<AES256>();
|
|
|
|
ctr->setKey(key.bytes, key.length);
|
|
}
|
|
}
|
|
/**
|
|
* Encrypt a packet
|
|
*
|
|
* @param bytes is updated in place
|
|
*/
|
|
virtual void encrypt(uint32_t fromNode, uint64_t packetId, size_t numBytes, uint8_t *bytes) override
|
|
{
|
|
if (key.length > 0) {
|
|
initNonce(fromNode, packetId);
|
|
if (numBytes <= MAX_BLOCKSIZE) {
|
|
static uint8_t scratch[MAX_BLOCKSIZE];
|
|
memcpy(scratch, bytes, numBytes);
|
|
memset(scratch + numBytes, 0,
|
|
sizeof(scratch) - numBytes); // Fill rest of buffer with zero (in case cypher looks at it)
|
|
|
|
ctr->setIV(nonce, sizeof(nonce));
|
|
ctr->setCounterSize(4);
|
|
ctr->encrypt(bytes, scratch, numBytes);
|
|
} else {
|
|
LOG_ERROR("Packet too large for crypto engine: %d. noop encryption!\n", numBytes);
|
|
}
|
|
}
|
|
}
|
|
|
|
virtual void decrypt(uint32_t fromNode, uint64_t packetId, size_t numBytes, uint8_t *bytes) override
|
|
{
|
|
// For CTR, the implementation is the same
|
|
encrypt(fromNode, packetId, numBytes, bytes);
|
|
}
|
|
|
|
private:
|
|
};
|
|
|
|
CryptoEngine *crypto = new STM32WLCryptoEngine();
|